Tag: apex
- Written by: ilmarkerm
- Category: Blog entry
- Published: February 23, 2025
To enable edit mode on Interactive Grid immediately when page loads (to save users a double click), you can create a dynamic action to run custom JavaScript on page load:
- Set Static ID on your Interactive Grid region – lets call it MYIG1
- Create a Dynamic Action on event Page Load and on True event let it execute the following JavaScript Code
apex.region("MYIG1").call("getActions").set("edit", true);
Up to this part all is well and if you page is not very log, problem solved. But if the page is longer you will notice a side-effect – the page gets automatically scrolled to set focus on the Interactive Grid. To fix it append a scroll up code to the JavaScript snippet above, so a full example would look like this.
apex.region("MYIG1").call("getActions").set("edit", true);
$("html, body").delay(500);
$("html, body").animate({ scrollTop: 0 },0);
That little bit of delay is necessary, otherwise scrolling will not work. It will also introduce a little bit of “page flickering”, but at least the page focus is back on top when page is loaded.
Tested with APEX 24.2.
- Written by: ilmarkerm
- Category: Blog entry
- Published: August 21, 2024
I previously wrote about how after successfully downgrading APEX users still get the error “Application Express is currently unavailable”. I now ran to the same issue again, with newer versions and the procedure how ORDS detects is APEX is currently being patched has changed.
This post is about downgrading to APEX 23.1 and ORDS 24.1.
After completing APEX downgrade to 23.1, ORDS 24.1 still reported that “Application Express is currently unavailable”. I followed my own previous blog and flipped the APEX internal patching status to APPLIED, but no luck this time. PANIC! I turned on tracking for ORDS sessions and this PL/SQL block seems to be the culprit.
DECLARE
NM OWA.VC_ARR := :1 ;
VL OWA.VC_ARR := :2 ;
L_PATCH VARCHAR2(10) := null;
L_VERSION VARCHAR2(100) := null;
SCHEMA VARCHAR2(30);
PART1 VARCHAR2(30);
PART2 VARCHAR2(30);
DBLINK VARCHAR2(30);
PART1_TYPE NUMBER(10);
OBJECT_NUM NUMBER(10);
BEGIN
OWA.INIT_CGI_ENV(:3 , NM, VL);
HTP.INIT;
HTP.HTBUF_LEN := 63;
--CHECK THE SCHEMA OVERRIDE FOR PL/SQL GATEWAY APPLICATION DETAILS
DECLARE
GATEWAY_DETAILS VARCHAR2(2000) := q'[ SELECT
(SELECT VALUE FROM ORDS_METADATA.USER_ORDS_PROPERTIES WHERE KEY = 'plsql.gateway.patching') as patchingValue,
(SELECT VALUE FROM ORDS_METADATA.USER_ORDS_PROPERTIES WHERE KEY = 'plsql.gateway.version') as versionValue
FROM dual]';
BEGIN
BEGIN
EXECUTE IMMEDIATE GATEWAY_DETAILS INTO L_PATCH, L_VERSION;
EXCEPTION
WHEN OTHERS THEN
-- ignore exception and leave l_patch as null so fallback is executed
NULL;
END;
IF L_PATCH IS NULL THEN
-- L_PATCH IS NULL, EITHER AN OLDER VERSION OF APEX IS PRESENT OR APEX IS NOT INSTALLED
-- CHECK IF APEX PRESENT
DECLARE
L_SQL VARCHAR2(200) := 'select patch_applied from apex_release';
BEGIN
DBMS_UTILITY.NAME_RESOLVE('APEX_RELEASE', 0, SCHEMA, PART1, PART2, DBLINK, PART1_TYPE, OBJECT_NUM);
EXECUTE IMMEDIATE L_SQL INTO L_PATCH;
EXCEPTION
WHEN OTHERS THEN
-- Could not access apex_release. Default l_patch to 'N' so it's still served
L_PATCH :='N';
END;
END IF;
END;
:4 := L_PATCH;
:5 :=L_VERSION;
END;
The flag that tells ORDS that APEX is currently being patched has been moved to ORDS_METADATA schema. And in my case the query indeed returned TRUE.
SELECT VALUE FROM ORDS_METADATA.USER_ORDS_PROPERTIES WHERE KEY = 'plsql.gateway.patching'
After flipping it to FALSE, downgraded APEX started working again.
UPDATE ORDS_METADATA.USER_ORDS_PROPERTIES set value='FALSE' WHERE KEY = 'plsql.gateway.patching';
- Written by: ilmarkerm
- Category: Blog entry
- Published: July 29, 2024
We are refreshing development databases from production using storage thin cloning. Everything works automatically and part of refresh procedure is also running some SQLPlus scripts, using OS authentication, as SYS.
One database also has APEX, that uses APEX Social Login feature to give users single sign-on for both APEX Builder and the end user application. You can read here how to set it up using Azure SSO. But since APEX is fully inside the database, this means that the production SSO credentials get copied over during database refresh.
I would like to have a SQL script that would run as part of the database refresh procedure, that would replace the APEX SSO credentials. And I’d like that script to run as SYS.
- Written by: ilmarkerm
- Category: Blog entry
- Published: July 18, 2024
We have been using Single-Sign On for APEX applications for a long time, way before there was social login feature available in APEX itself. We implemented it on web server level in front of APEX. But in order to simplify the webserver setup (mainly to disable sticky load balancer setup) and since APEX for quite some time can do some SSO protocols now internally – we finally moved to APEX social sign-in – using OpenID connector. I blogged about it here earlier.
Enabled it last evening, everything worked fine over night (low use), and suddenly, this morning, when the real use begins, application users started getting the following login failures:
The HTTP request to “https://login.microsoftonline.com/<tenancy_id>/v2.0/.well-known/openid-configuration” failed.
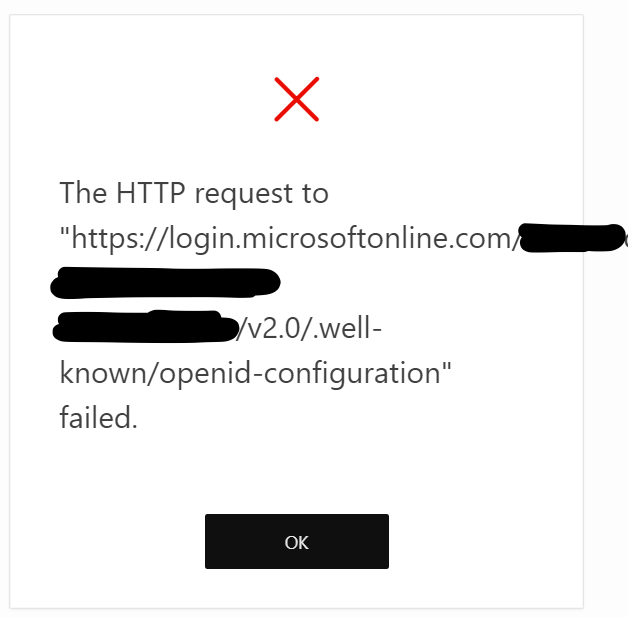
Quite un-informative… why did it fail? This URL is the OpenID discovery URL and the message comes from APEX, not Azure SSO. A few quick checks:
- The URL works, and it returns proper 200 OK status code, even from the database server
- If there were any TLS errors, then the error message would be different
- No new messages in APEX_DEBUG_MESSAGES since the issue started
- Nothing in APEX_WEBSERVICE_LOG
- Nothing in alert.log 🤔
- Changing APEX instance proxy did not help
- TLS wallet was accessible and good
- The error is returned ONLY to application users, even though builder uses the same OpenID Discovery URL – and APEX builder login works without any issues
- And every developer swore, that noone has changed anything today
As a side-note – the end-user applications are quite heavily used and there are hundreds of internal users.
Finally I started creating new Authentications scheme using Generic OAuth2 instead and they seemed to connect to Azure services without issues. But then messages started appearing in APEX_DEBUG_MESSAGES (what triggered that DEBUG logging started writing? accessing applications via Builder?) and hidden deep in messages I found the gem:
Exception in "GET https://login.microsoftonline.com/azure_tenancy_id/v2.0/.well-known/openid-configuration":
Error Stack: ORA-20001: You have exceeded the maximum number of web service requests per workspace. Please contact your administrator.
Looks like, at least in APEX 23.1, calls to OpenID discovery URL count towards the APEX workspace web service request limiter, after increasing the limit the authentication started working again.
exec APEX_INSTANCE_ADMIN.SET_PARAMETER('MAX_WEBSERVICE_REQUESTS', 100000);
Problem solved, but it was quite confusing to troubleshoot due to lack of more detailed error messages. I really do not expect authentication hitting a rate limiter and then being completely silent about the underlying cause.
- Written by: ilmarkerm
- Category: Blog entry
- Published: October 28, 2023
JSON Web Token (JWT) is a popular open standard that enables web applications to transfer information between parties asn JSON object. For example single-sign on authentication information. The information can be trusted, because the token also includes a signature. Verifying this signature is essential, otherwise anyone can fake the token contents.
Oracle APEX does provide APEX_JWT package, which handles the parsing and validity checking very well, but it can only verify the signature, if JWT was created using HS256 signature. HS256 is loosing popularity, since it is based on symmetric cryptography, meaning all parties must have access to the same encryption key.
Another, much more secure signature is now gaining popularity, based on RSA public key encryption – RS256. Here JWT is signed using a private key, but it can be verified using the corresponding public key. As the name suggests, public key is completely public and can be downloaded from the internet using kid attribute value present in JWT header (this service is called JWKS – JSON Web Key Sets). This is also the signature system AWS Cognito uses.
At the time of writing (APEX 23.1 and Oracle database 19.20), I did not find and ready code on the internet for verifying JWT RS256 signatures – so I had to create one. It lets APEX_JWT do the JWT parsing and validity checking, but I needed to add RS256 signature checking and downloading keys from JWKS store. It is intended to be used from APEX flows.
APEX_JWT_RS256 package repository can be found here
A quick example how to use the package as APEX page sentry function in custom authentication scheme.
create or replace FUNCTION JWT_PAGE_SENTRY RETURN BOOLEAN AS
v_required_group varchar2(30):= 'important_people'; -- Group needed to access the app
v_iss varchar2(200):= 'https://cognito-idp.eu-central-1.amazonaws.com/eu-central-1_ZZxxZZxx11'; -- ISS that issued the JWT, YOU MUST CHANGE THIS to point to your own ISS
jwt_cookie owa_cookie.cookie;
v_jwt_payload varchar2(2000);
v_jwt_json json_object_t;
v_groups json_array_t;
v_group_found boolean:= false;
BEGIN
-- Do JWT token validation and check that correct group is granted to user
-- 2023 Ilmar Kerm
jwt_cookie:= owa_cookie.get('JWT_COOKIE_NAME');
IF jwt_cookie.vals.COUNT = 0 THEN
apex_debug.error('JWT session cookie not found');
RETURN false;
END IF;
IF apex_jwt_rs256.decode_and_validate(jwt_cookie.vals(1), v_iss, v_jwt_payload) THEN
-- JWT validated, now check the required group
v_jwt_json:= json_object_t.parse(v_jwt_payload);
v_groups:= v_jwt_json.get_array('cognito:groups');
FOR i IN 0..v_groups.get_size - 1 LOOP
IF v_groups.get_string(i) = v_required_group THEN
v_group_found:= true;
EXIT;
END IF;
END LOOP;
IF NOT v_group_found THEN
apex_debug.error('Required group is missing from JWT: '||v_required_group);
RETURN false;
END IF;
IF v_jwt_json.get_string('token_use') != 'access' THEN
apex_debug.error('Invalid value for JWT attribute token_use');
RETURN false;
END IF;
IF V('APP_USER') IS NULL OR V('APP_USER') = 'nobody' OR V('APP_USER') != v_jwt_json.get_string('username') THEN
APEX_CUSTOM_AUTH.DEFINE_USER_SESSION(
p_user => v_jwt_json.get_string('username'),
p_session_id => APEX_CUSTOM_AUTH.GET_NEXT_SESSION_ID
);
END IF;
RETURN true;
ELSE
RETURN false;
END IF;
END JWT_PAGE_SENTRY;