Building Oracle Cloud infrastructure with Terraform – Basics
- Written by: ilmarkerm
- Category: Blog entry
- Published: March 24, 2024
I thought I’ll start exploring Oracle Cloud offerings a little and try building something with Terraform.
The execution environment
OCI Could Console offers Cloud Shell and Code Editor right from the browser. Cloud Shell is a small Oracle Linux container with shell access, that has the most popular cloud tools and OCI SDKs already deployed. Most importantly, however, all Oracle Cloud API commands you execute from there, they run silently as yourself, no additional setup required. Including setting up terraform. Pretty awesome idea I would say – no need to set up any admin computer first.
Since I would mainly write code, I’m going to use only only Code Editor (which is actually VS Code in your browser) and VS Code also has a built in terminal for executing commands.
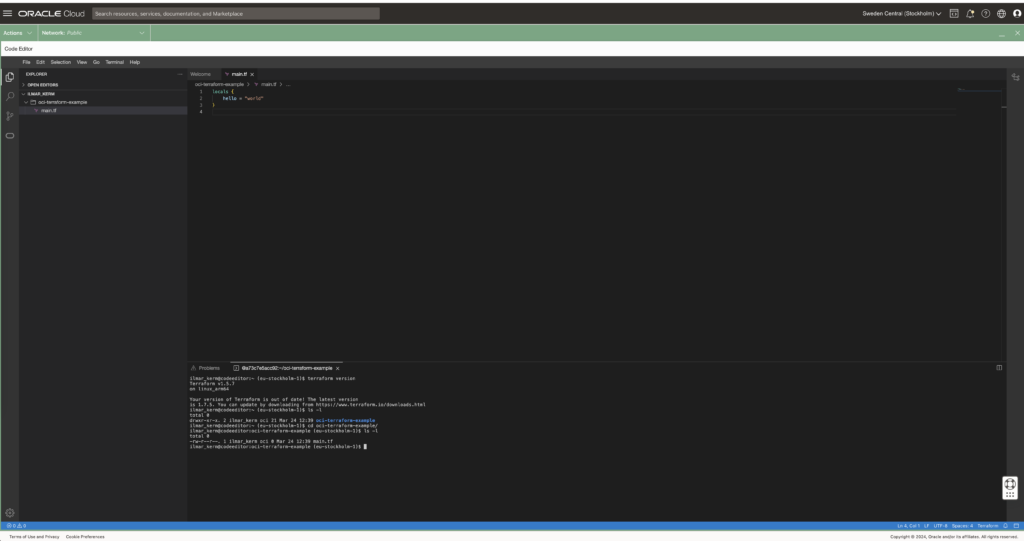
Read about executing and using Cloud Shell here.
Setting up Terraform provider
When executing from Cloud Shell / Code Editor, then setting up the terraform provider is very simple.
# versions.tf
provider "oci" {
region = "eu-stockholm-1"
}
It is very good practice to also place terraform state file in the shared object store. OCI also provides an object store and to set it up first create a Bucket in Object Storage.
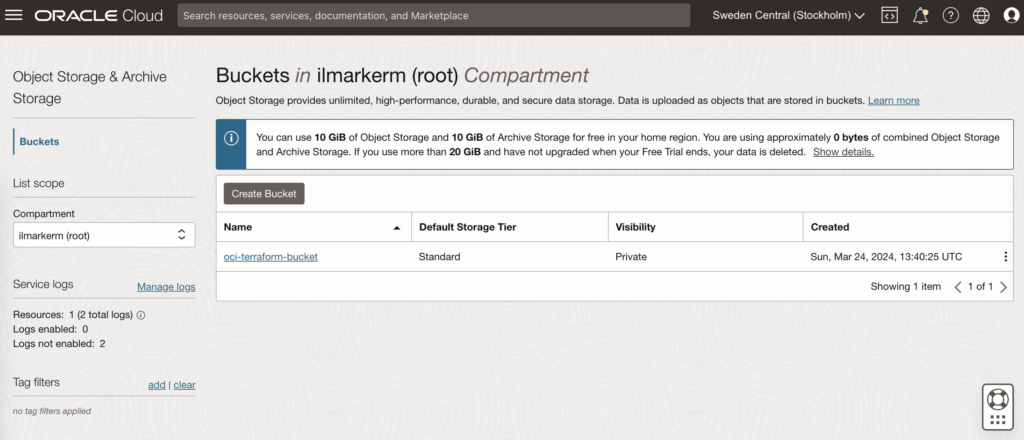
This also requires setting up Customer Secret Keys, for accessing the bucket using S3 protocol. I’m going to save my access key and secret access key in a file named bucket.credentials.
# bucket.credentials
[default]
aws_access_key_id=here is your access key
aws_secret_access_key=here is your secret access key
# remote_state.tf
terraform {
backend "s3" {
bucket = "oci-terraform-bucket"
key = "oci-terraform.tfstate"
region = "eu-stockholm-1"
# ax9u97qgbo5h is the namespace of the bucket, it is shown in the Bucket Details page
endpoint = "https://ax9u97qgbo5h.compat.objectstorage.eu-stockholm-1.oraclecloud.com"
shared_credentials_file = "bucket.credentials"
skip_region_validation = true
skip_credentials_validation = true
skip_metadata_api_check = true
force_path_style = true
}
}
Creating compartment and basic networking
Compartment is just a handy hierarchical logical container which helps to organise your Oracle Cloud resources better. It can also be used to set common tags for all resources created under it.
# main.tf
locals {
tenancy_id = "ocid1.tenancy.oc1..aaaaaaaawf2fv3ipfdp564ffiqpfqr6u6n3uofydgtihq3wget5357lq5i6a"
environment = "dev"
}
# Information about current tenancy, for example home region
data "oci_identity_tenancy" "tenancy" {
tenancy_id = local.tenancy_id
}
# Get the parent compartment as a terraform object
data "oci_identity_compartment" "parent_compartment" {
# Top get list of existing compartments execute:
# oci iam compartment list
id = data.oci_identity_tenancy.tenancy.id
}
# Create compartment
resource "oci_identity_compartment" "compartment" {
# Compartment_id must be the parent compartment ID and it is required
compartment_id = data.oci_identity_compartment.parent_compartment.id
description = "oci-terraform experiments"
name = "oci-terraform-experiments"
# Define some default tags that are added to all resources created under this compartment
freeform_tags = {
"deployed_by" = "terraform"
"environment" = local.environment
}
}
To set up networking, first you need VCN Virtual Cloud Network and under it subnets.
# network.tf
resource "oci_core_vcn" "main" {
compartment_id = oci_identity_compartment.compartment.id
display_name = "VCN for oci-terraform test"
dns_label = "ocitf"
cidr_blocks = ["10.1.2.0/24"]
is_ipv6enabled = false
}
resource "oci_core_subnet" "subnet" {
cidr_block = "10.1.2.0/25"
compartment_id = oci_identity_compartment.compartment.id
vcn_id = oci_core_vcn.main.id
# List availability domains
# oci iam availability-domain list
# Documentation recommends creating regional subnets instead, without specifying availability_domain
#availability_domain = "MpAX:EU-STOCKHOLM-1-AD-1"
display_name = "Subdomain #1"
prohibit_internet_ingress = false
prohibit_public_ip_on_vnic = false
}
To be continued
I don’t really know where this post series is going. I’ve done quite a bit of Terraforming in AWS, so here I’m just exporing what Oracle Cloud has to offer and instead of using the dreaded ClickOps, I’ll try to be proper with Terraform.
At the end of the post I have these resources created.
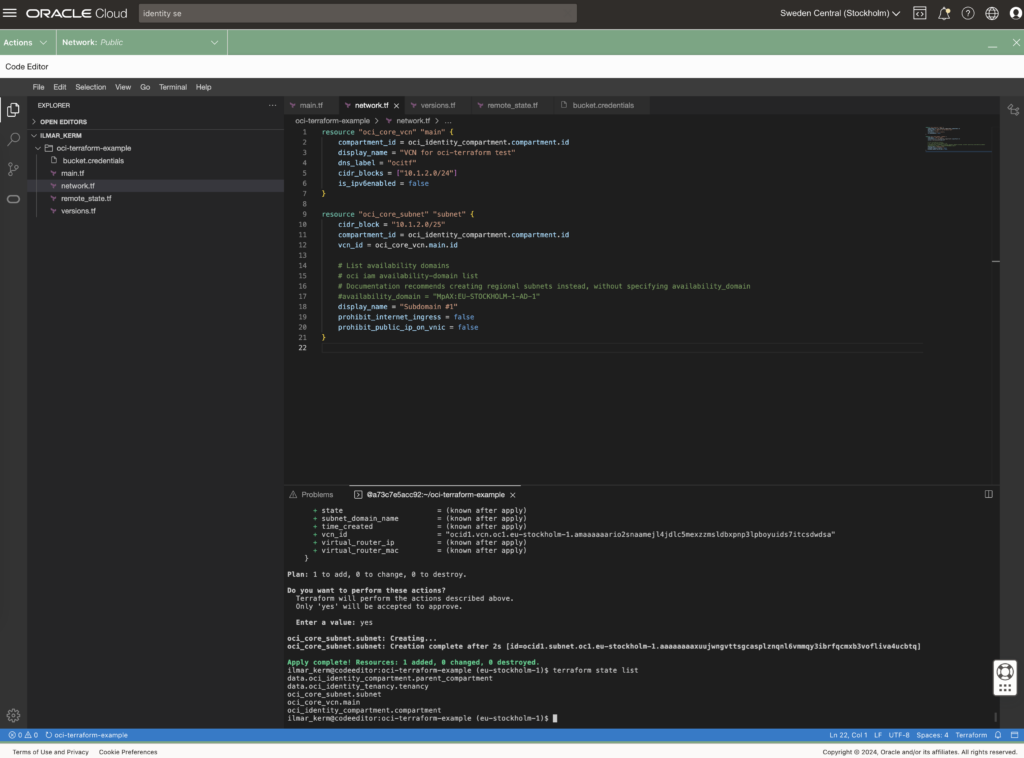